1. Introduction
K-Means clustering is a widely-used algorithm in the field of data analysis and machine learning, designed to partition a dataset into distinct groups or clusters based on similarity. This method is particularly valuable in identifying patterns and structures within data, making it a powerful tool in various applications such as market segmentation, image processing, and anomaly detection. In this guide, we will explore K-Means clustering in detail, walking through the algorithm step-by-step and providing practical insights to help you effectively implement it.
2. Understanding K-Means Clustering
2.1. How K-Means Works
K-Means clustering works by dividing a dataset into K clusters, where each data point belongs to the cluster with the nearest mean (centroid). The algorithm iteratively refines the positions of the centroids until the assignments stabilize.
2.2. Key Terminology
- Clusters: Groups of data points that are similar to each other.
- Centroids: The center point of a cluster, calculated as the mean of all data points in that cluster.
- Iterations: The repeated process of assigning data points to clusters and updating centroids.
2.3. The K-Means Algorithm Steps
The K-means algorithm consists of a few key steps: initialization, assignment of points to clusters, centroid update, and iteration until convergence.
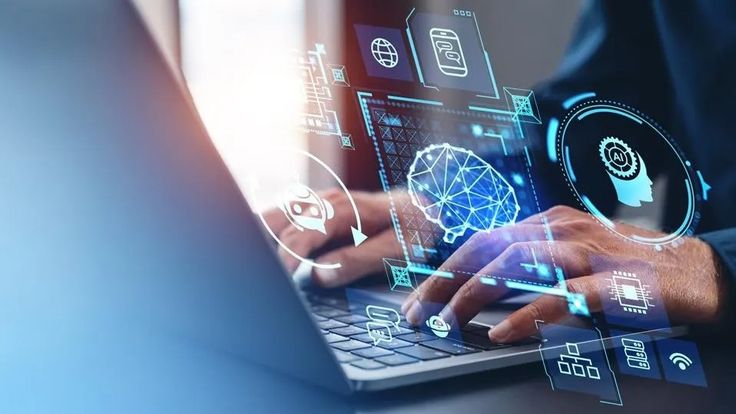
3. Step-by-Step Guide to K-Means Clustering
3.1. Step 1: Data Preparation
Before implementing K-Means, it’s crucial to prepare your data:
- Data Cleaning: Handle missing values and outliers to ensure the dataset is reliable.
- Feature Selection: Choose relevant features that contribute meaningfully to the clustering process.
- Scaling: Normalize or standardize your data, especially if features have different units or scales, to avoid bias in distance calculations.
3.2. Step 2: Choose the Number of Clusters (K)
Determining the optimal number of clusters (K) is essential for effective segmentation. Common methods include:
- Elbow Method: Plot the explained variance against the number of clusters and look for the “elbow” point, where adding more clusters yields diminishing returns.
- Silhouette Score: Measure how similar an object is to its own cluster compared to other clusters. A higher silhouette score indicates a better-defined cluster.
3.3. Step 3: Initialize Centroids
Choose initial centroids for the clusters. Common methods include:
- Random Selection: Randomly pick K data points as initial centroids.
- K-Means++: An advanced method that selects initial centroids in a way that improves the convergence speed and final results.
3.4. Step 4: Assign Data Points to Clusters
For each data point, calculate its distance from each centroid and assign it to the nearest cluster. The most common distance metric used is Euclidean distance, but you can choose others based on your data’s characteristics.
3.5. Step 5: Update Centroids
Once all data points have been assigned to clusters, recalculate the centroids by taking the mean of all data points in each cluster. This new position represents the updated centroid.
3.6. Step 6: Iterate Until Convergence
Repeat the assignment and update steps until the centroids no longer change significantly, indicating that the algorithm has converged. You can also set a maximum number of iterations to avoid infinite loops.
4. Evaluating K-Means Clustering
4.1. Evaluating Cluster Quality
Evaluate the quality of your clusters using metrics such as:
- Within-Cluster Sum of Squares (WCSS): Measures the compactness of the clusters. Lower values indicate better clustering.
- Silhouette Score: As mentioned earlier, it quantifies how similar a data point is to its own cluster versus other clusters.
4.2. Visualizing Clusters
Visual representation helps understand clustering results. Techniques include:
- Scatter Plots: Plotting data points colored by cluster assignment.
- PCA: Use Principal Component Analysis to reduce dimensionality for visualization purposes.
4.3. Interpreting Results
Interpret the results by analyzing the characteristics of each cluster, understanding the common traits of data points within clusters, and deriving actionable insights.
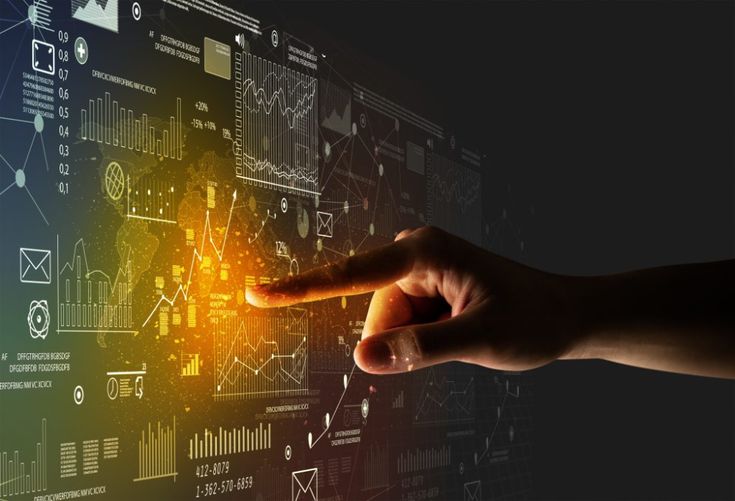
5. Practical Applications of K-Means Clustering
5.1. Market Segmentation
Businesses use K-Means clustering to segment customers into distinct groups based on purchasing behavior, enabling targeted marketing strategies.
5.2. Image Compression
K-Means can be applied to reduce the number of colors in an image, effectively compressing it while preserving its quality.
5.3. Anomaly Detection
By clustering normal data points, K-Means can help identify outliers that deviate significantly from established patterns.
6. Challenges and Limitations of K-Means Clustering
6.1. Sensitivity to Initialization
The choice of initial centroids can significantly affect the final clustering results. Running the algorithm multiple times with different initializations can help mitigate this issue.
6.2. Choosing the Right K
Determining the optimal number of clusters can be challenging and may require domain knowledge or experimentation.
6.3. Assumption of Spherical Clusters
K-Means assumes clusters are spherical and equally sized. This assumption can lead to poor clustering results if the actual data structure does not conform to these characteristics.
7. Tools and Libraries for K-Means Clustering
7.1. Popular Libraries
Several libraries facilitate K-Means clustering:
- Scikit-learn: A Python library offering a robust implementation of the K-Means algorithm.
- R: The
stats
package provides a straightforward implementation of K-Means. - MATLAB: Offers built-in functions for clustering, including K-Means.
7.2. Step-by-Step Implementation Guide
Here’s a basic implementation of K-Means using Scikit-learn in Python:
import numpy as np
import pandas as pd
from sklearn.cluster import KMeans
import matplotlib.pyplot as plt
# Step 1: Data Preparation
data = pd.read_csv('data.csv') # Load your data
features = data[['feature1', 'feature2']] # Select features
features = (features - features.mean()) / features.std() # Scale features
# Step 2: Choose the number of clusters (K)
wcss = []
for i in range(1, 11):
kmeans = KMeans(n_clusters=i)
kmeans.fit(features)
wcss.append(kmeans.inertia_)
# Plot the Elbow Method
plt.plot(range(1, 11), wcss)
plt.title('Elbow Method')
plt.xlabel('Number of clusters')
plt.ylabel('WCSS')
plt.show()
# Step 3: Initialize K-Means with optimal K
optimal_k = 3 # Assuming 3 from Elbow Method
kmeans = KMeans(n_clusters=optimal_k)
clusters = kmeans.fit_predict(features)
# Step 4: Visualize clusters
plt.scatter(features['feature1'], features['feature2'], c=clusters)
plt.scatter(kmeans.cluster_centers_[:, 0], kmeans.cluster_centers_[:, 1], s=300, c='red', label='Centroids')
plt.title('K-Means Clustering')
plt.xlabel('Feature 1')
plt.ylabel('Feature 2')
plt.legend()
plt.show()
8. Conclusion
K-Means clustering is a versatile and efficient method for segmenting data into distinct groups based on similarity. By following the step-by-step process outlined in this guide, you can effectively implement K-Means clustering in your data analysis projects. Whether for market segmentation, image processing, or anomaly detection, mastering K-Means can significantly enhance your analytical capabilities and provide valuable insights.
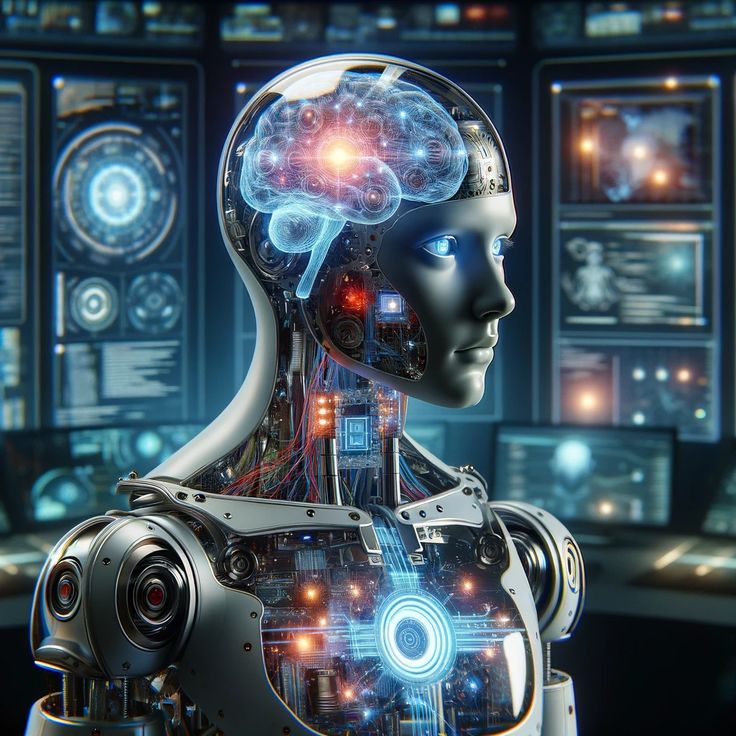
FAQs about K-Means Clustering
1. What is K-Means clustering?
- K-Means clustering is an unsupervised machine learning algorithm used to partition a dataset into K distinct clusters based on similarity. Each data point is assigned to the cluster with the nearest centroid.
2. How do I determine the optimal number of clusters (K)?
- The optimal number of clusters can be determined using methods like the Elbow Method, where you plot the Within-Cluster Sum of Squares (WCSS) against the number of clusters and look for the “elbow” point, or the Silhouette Score, which measures how similar a data point is to its own cluster versus other clusters.
3. What distance metric is commonly used in K-Means clustering?
- The most commonly used distance metric is Euclidean distance. However, you can also use other metrics, such as Manhattan distance or Cosine similarity, depending on your data.
4. What are the limitations of K-Means clustering?
- K-Means clustering is sensitive to the initial choice of centroids, requires specifying the number of clusters in advance, assumes spherical clusters of equal size, and can be affected by outliers.
5. How can I improve the performance of K-Means clustering?
- To improve performance, you can try using the K-Means++ initialization method to select better initial centroids, scale your features, experiment with different distance metrics, or combine K-Means with other clustering techniques.
6. Can K-Means be used for non-numeric data?
- K-Means is primarily designed for numeric data due to its reliance on distance calculations. However, you can preprocess categorical data using techniques like one-hot encoding before applying K-Means.
7. What industries commonly use K-Means clustering?
- K-Means clustering is widely used in various industries, including retail for customer segmentation, finance for risk assessment, healthcare for patient classification, and marketing for targeted campaigns.
8. How do I visualize the results of K-Means clustering?
- You can visualize the results using scatter plots with different colors representing different clusters. Dimensionality reduction techniques like PCA can also be employed to visualize clusters in two dimensions.
9. What tools or libraries can I use for implementing K-Means clustering?
- Popular tools include Python’s Scikit-learn, R’s
stats
package, and MATLAB, all of which offer built-in functions for K-Means clustering.
10. How can I interpret the results of K-Means clustering?
- Analyze the characteristics of each cluster by examining the mean or median values of the features within each cluster. This analysis can provide insights into customer behaviors or trends in the data.
Tips for Effective Use of K-Means Clustering
- Preprocess Your Data: Clean your dataset by handling missing values and outliers, and consider normalizing or standardizing your features to ensure consistent scales.
- Experiment with Different Values of K: Try multiple values for K and evaluate the clustering results to find the most suitable number of clusters for your specific dataset.
- Use K-Means++ Initialization: Implement the K-Means++ method for initializing centroids to improve convergence speed and avoid poor clustering outcomes.
- Monitor Cluster Quality: Regularly assess the quality of your clusters using metrics such as WCSS and Silhouette Score, and adjust your approach as needed.
- Visualize Your Data: Create visualizations to better understand the structure of your clusters and effectively communicate your findings to stakeholders.
- Combine with Other Techniques: Consider combining K-Means with other clustering methods or techniques like hierarchical clustering to gain deeper insights from your data.
- Be Aware of Limitations: Understand the limitations of K-Means clustering, including its sensitivity to outliers and the assumption of spherical clusters, and be prepared to address these challenges.
- Iterate on Your Model: Don’t hesitate to iterate on your clustering model based on the insights gained. Adjust your features or the number of clusters to refine your results.
- Stay Informed: Keep learning about advancements in clustering algorithms and techniques to enhance your analysis and stay updated with best practices.
- Document Your Process: Maintain detailed documentation of your K-Means clustering process, including data preparation steps, parameters used, and insights gained, to inform future projects.